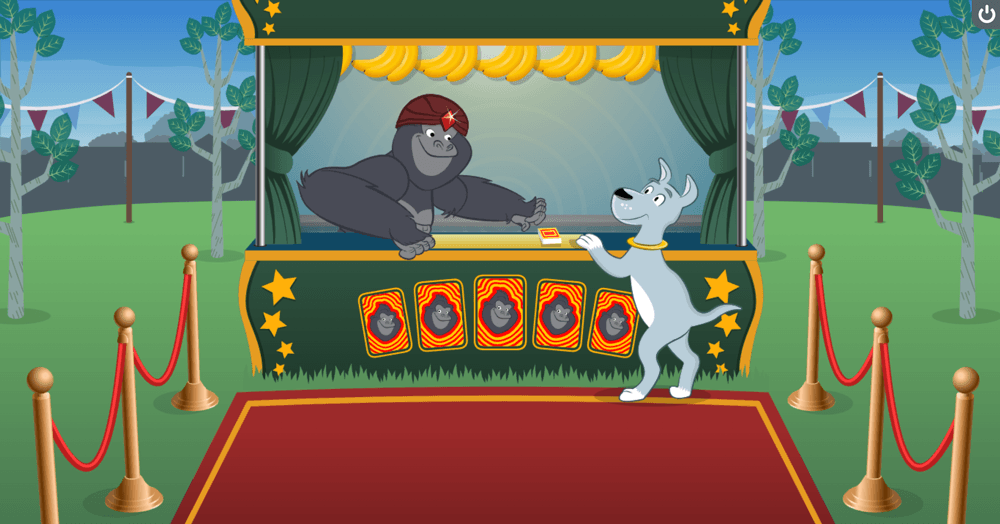
Ambrasoft is a suite of educational maths and language games owned by the Dutch company, Noordhoff. Back in 2015, whilst working at LJ Create, I was part of a project team that converted a large collection of Flash games to HTML Canvas, along with creating new games from scratch.
I was responsible for writing the Javascript logic, drawing vector assets and creating sound effects on several of the games. We used the Canvas library CreateJS, a library which allowed us to create rich interactive content that performed well on a range of devices.
Gorillarama (new game)
A matching pairs game where the player goes up against an AI gorilla. The player has to find more pairs than the gorilla to advance to the next round, with each round getting progressively harder.
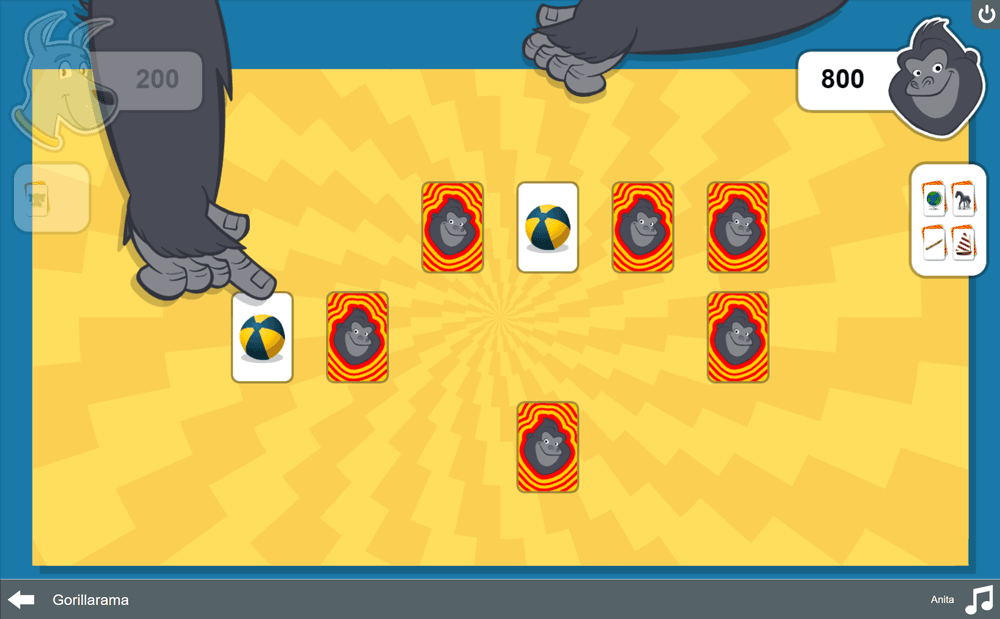
The harder rounds have a larger grid of cards and the gorilla has a better memory of which cards he has already seen, making him more likely to pick correct pairs.
function gregoryPickSecondCard() {
var oSecondCard = null,
fMemoryPickBias = oRoundProps[iCurrentRoundNumber].fGregCheckFromMemoryBias,
bAttemptPickFromMemory = getBiasedBoolean(fMemoryPickBias);
if (bAttemptPickFromMemory) {
// Search for match in greg's memory
oSecondCard = getMatchingCardFromGregsMemory(oGregsFirstCard);
}
if (!oSecondCard) {
// Get random second card
// Exclude first card
var oaCardsToExclude = [oGregsFirstCard];
if (bAttemptPickFromMemory) {
// Match not found in gregory's memory, exclude memory cards
oaCardsToExclude.concat(oaGregorysCardMemory);
}
oSecondCard = getRandomTableCard(oaCardsToExclude);
}
// Play card animation
playGregoryPointAnimation(oSecondCard);
}
Brick Donk (new game)
A breakout clone that uses vector assets from the Ambrasoft library. I created the game engine in JavaScript. It managed ball physics, collisions, dynamic drawing, powerups (extra wide bat, shield, slow ball speed, fireball) and the scoring system.
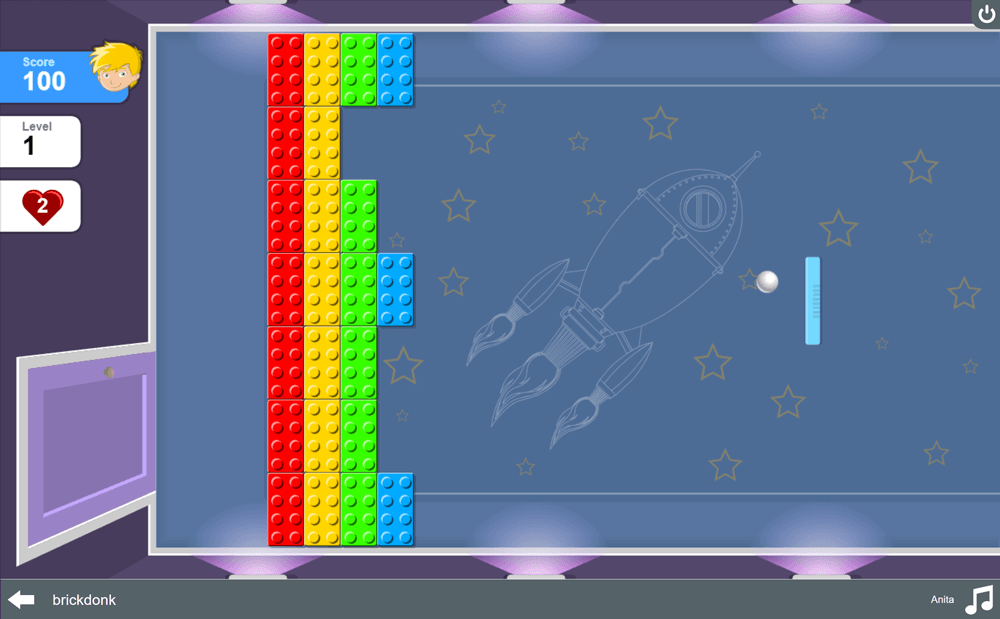
function drawMultipleFillColoursIntoBrick(oFill, aoaColours) {
var oFillContainer = oFill,
iNumRows = aoaColours.length,
iNumColumns = aoaColours[0].length,
iMaxIndexX = iNumColumns - 1,
iMaxIndexY = iNumRows - 1;
for (var i = 0; i < aoaColours.length; i++) {
for (var k = 0; k < aoaColours[i].length; k++) {
// Gather props
var sColour = getRGBAString(aoaColours[i][k]),
iRadTL = (i === 0 && k === 0) ? BRICK_CORNER_RAD:0,
iRadTR = (i === 0 && k === iMaxIndexX) ? BRICK_CORNER_RAD:0,
iRadBR = (i === iMaxIndexY && k === iMaxIndexX) ? BRICK_CORNER_RAD:0,
iRadBL = (i === iMaxIndexY && k === 0) ? BRICK_CORNER_RAD:0;
// Draw new fill
var oFillShape = new createjs.Shape();
oFillShape.graphics.beginFill(sColour);
oFillShape.graphics.drawRoundRectComplex(0, 0, BRICK_HUB_SIZE, BRICK_HUB_SIZE, iRadTL, iRadTR, iRadBR, iRadBL);
oFillShape.graphics.endFill();
// Position
oFillShape.x = k * BRICK_HUB_SIZE;
oFillShape.y = i * BRICK_HUB_SIZE;
// Add fill to container
oFillContainer.addChild(oFillShape);
}
}
}
Word Search (converted game)
A simple word search where the player uses a click and drag mechanism to find 10 words on the grid. Correct guesses trigger positive feedback and incorrect responses trigger negative feedback. The game ends when all of the words have been found.
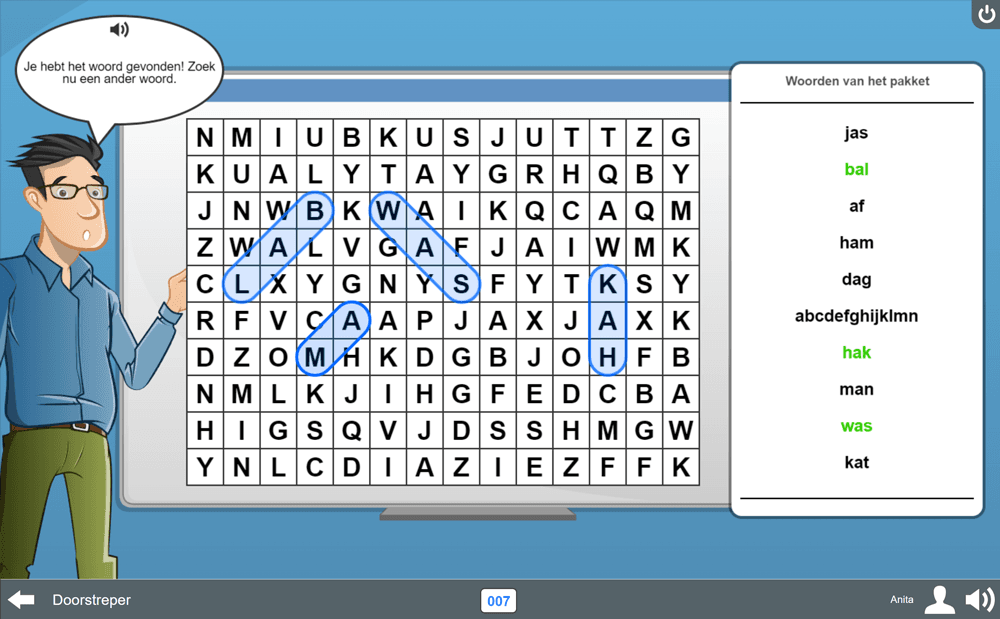
The words are pushed into a 2d grid array in random directions. The attempted string is calculated using the XY coordinates of the highlight shape start and end points.
function getAttemptedString() {
var sResult = "",
iXDiff = oHighlightIndices.oStart.x - oHighlightIndices.oEnd.x,
iYDiff = oHighlightIndices.oStart.y - oHighlightIndices.oEnd.y;
if (iXDiff != 0 && iYDiff != 0 && forcePositive(iXDiff) != forcePositive(iYDiff)) {
// invalid
} else {
var iWordLength = Math.max(forcePositive(iXDiff), forcePositive(iYDiff)) + 1,
iXIndex = oHighlightIndices.oStart.x,
iYIndex = oHighlightIndices.oStart.y,
iXDir = getDirectionFromDifference(iXDiff),
iYDir = getDirectionFromDifference(iYDiff);
for (var i = 0; i < iWordLength; i++) {
sResult += aoaGrid[iXIndex][iYIndex];
iXIndex += iXDir;
iYIndex += iYDir;
}
}
return sResult;
}
Technologies Used:
- JavaScript
- CreateJS
- Adobe Animate
- Illustrator
- SCSS